A step-by-step guide for Smart Contract deployment using Hardhat

Posted By
Anamol Soman
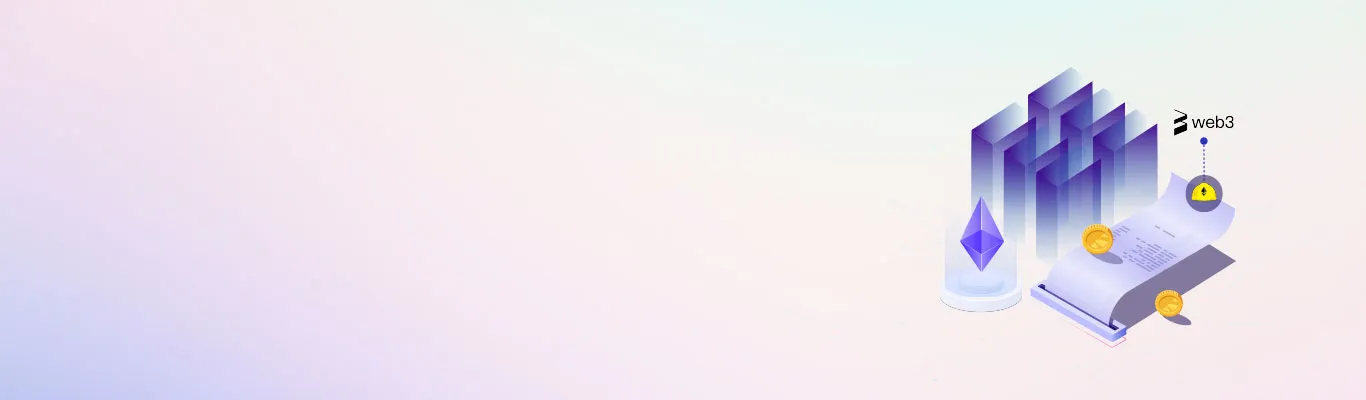
Blog 2/3 of our series focused on Web3 is here. If you're getting into Ethereum development, one of the first things you'll probably want to do is deploy a Smart Contract. And trust me, it's not as daunting as it sounds! In this blog, I'm going to walk you through the steps to deploy a Smart Contract on the Sepolia Ethereum testnet using Hardhat.
If you're unfamiliar with it, Sepolia is a test network where you can deploy contracts without using real Ether—perfect for testing and experimenting. Let's break it down step-by-step. By the end, you'll have your first contract running on Sepolia and be one step closer to becoming an Ethereum developer!
Prerequisites for deploying a Smart Contract
Before we start, you’ll need a few things ready:
- MetaMask Wallet: You'll need MetaMask to interact with Ethereum and sign transactions. You can download the browser extension from here. Ensure you've also got some Sepolia ETH in your wallet (more on this in a second).
- Sepolia ETH: Since Sepolia is a testnet, you need Sepolia ETH to pay for gas fees. You can easily get some by visiting a Sepolia faucet online. Your MetaMask wallet must be connected to the faucet to get the ETH.
- Node.js: Hardhat runs on Node.js, so you need it installed. If you don't have it, download it from here.
- Hardhat: This development framework makes deploying Smart Contracts super easy. We'll install it next!
Steps to Deploy a Smart Contract with Hardhat
Streamline your deployment process. Follow these straightforward steps to get your Smart Contract live
Step 1: Set up your Hardhat project
Let's set up your Hardhat project. Open your terminal (or command prompt) and follow these steps:
1. Create a new directory for your project. This keeps everything organized.
mkdir MyHardhatProject cd MyHardhatProject
2. Initialize a new Node.js project. This step generates a package.json file, essential for managing your dependencies.
npm init -y
3. Install Hardhat as a development dependency.
npm install --save-dev hardhat
4. Initialize the Hardhat project now that Hardhat is installed.
npx hardhat
When you run this command, Hardhat will ask you a few questions. Don't worry about anything complicated; choose "Create a basic sample project" and hit Enter. This will set up a basic project with some sample code to get you started.
Step 2: Install the dependencies for Sepolia and Hardhat
Now that we have a basic Hardhat project, we need a few extra tools to help us deploy the contract to Sepolia.
Run this command to install everything we need:
npm install @nomiclabs/hardhat-ethers ethers @nomiclabs/hardhat-waffle
Here’s what these packages do:
- @nomiclabs/hardhat-ethers: This plugin allows Hardhat to work with the Ethers.js library, which is super useful for interacting with Ethereum.
- ethers: This JavaScript library lets you connect to the Ethereum blockchain and send transactions.
- @nomiclabs/hardhat-waffle: This gives us testing tools that make writing tests for your Smart Contracts easy (we'll use this later when you're ready to test contracts).
Step 3: Set up the Sepolia network configuration
We're almost there! Now, let's configure Hardhat to work with the Sepolia test network.
1. In your project folder, find the hardhat.config.js file and open it.
2. We need to add a configuration for Sepolia so Hardhat knows how to connect to it. Replace the contents of hardhat.config.js with the following:
require('@nomiclabs/hardhat-ethers'); module.exports = { solidity: "0.8.19", // Make sure to match the version of Solidity you're using networks: { sepolia: { url: 'https://sepolia.infura.io/v3/YOUR_INFURA_PROJECT_ID', // Get your Infura API Key accounts: [`0x${process.env.PRIVATE_KEY}`] // Your MetaMask private key (use .env to secure this) }, }, };
Here’s the breakdown:
- solidity: Specifies the version of Solidity we're using for the contract.
- networks: Configures the networks where you can deploy your contracts. We add the Sepolia network here, with the Infura URL and your wallet's private key.
- To connect to Sepolia, you'll need an Infura account. Go to Infura and create an account. Then, create a new project and grab your API key.
- For security, never hardcode your private key directly into the configuration file. Instead, use environment variables (.env file).
Step 4: Write a simple Smart Contract
Now, let's write the actual Smart Contract that we'll deploy.
Inside your project folder, go to the contracts directory. You should see a file called Greeter.sol. You can edit this to create a simple contract, or create a new one.
Here’s a basic example of a simple contract:
// SPDX-License-Identifier: MIT pragma solidity ^0.8.19; contract MyFirstContract { string public greeting = "Hello, Sepolia!"; function setGreeting(string memory _greeting) public { greeting = _greeting; } }
This contract simply stores a greeting message and lets you update it.
Step 5: Deploy the Contract to Sepolia
Now the fun part: deployment!
1. Inside the scripts directory, you should see a sample-script.js file. You can replace its contents with the following script to deploy your contract:
async function main() { const [deployer] = await ethers.getSigners(); console.log("Deploying contracts with the account:", deployer.address); const Contract = await ethers.getContractFactory("MyFirstContract"); const contract = await Contract.deploy(); console.log("Contract deployed to address:", contract.address); } main() .then(() => process.exit(0)) .catch((error) => { console.error(error); process.exit(1); });
2. Before running this, make sure you’ve set up your private key in an .env file. You can use the dotenv package to load the private key securely.
3. Once you’re ready, run the deployment script using:
npx hardhat run scripts/deploy.js --network sepolia
Hardhat will deploy your contract to the Sepolia testnet, and you should see the contract's address printed out in the console!
Step 6: Interact with your contract
Congrats! Your contract is now live on Sepolia. You can interact with it by calling its functions using Hardhat or through a web interface like Etherscan or MetaMask.
If you want to interact programmatically with your deployed contract, you can use Hardhat scripts or build a front-end with Web3 or Ethers.js to interact with the contract in your browser. To familiarize yourself with Web3, read our previous blog that explains the basics of Web3 and how its seen as the future of the internet.
Key takeaway
Using Hardhat, you've just deployed your first Smart Contract to the Sepolia test network. From here, you can experiment with more advanced contracts, write tests, and eventually deploy to the Ethereum mainnet. Remember, the key takeaway is that Hardhat makes Ethereum development much more manageable by streamlining the process of compiling, deploying, and testing contracts. With Sepolia as your testnet, you can experiment freely without the worry of losing real ETH. Happy coding, and good luck with your Ethereum journey!
Related Blogs
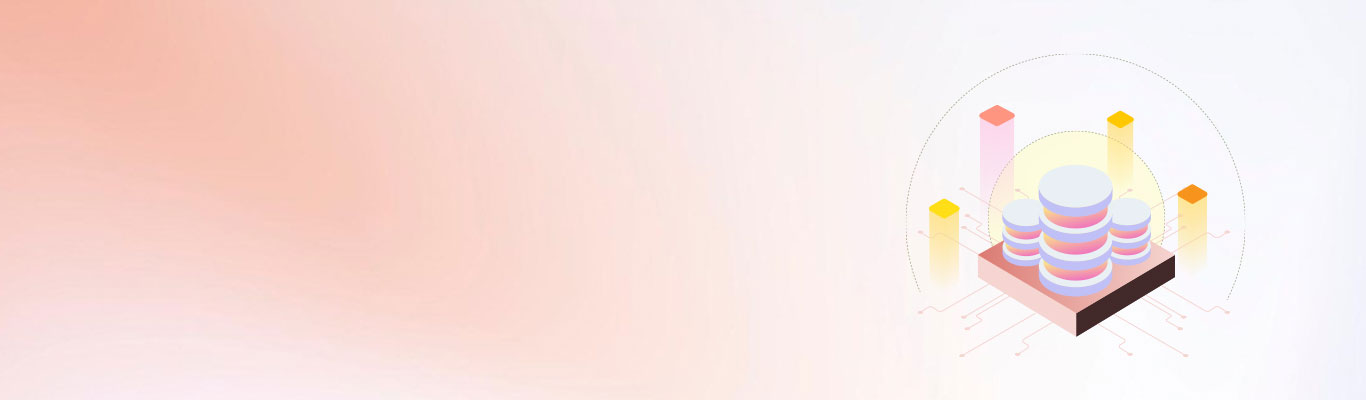
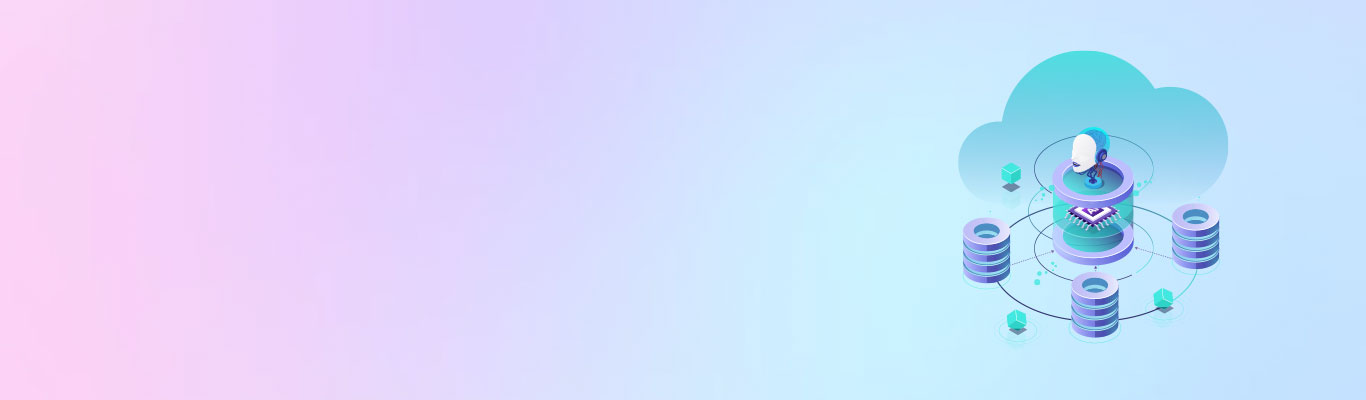